Explore The 7 JavaScript Concepts Every Web Developer Should Know
JavaScript is a programming language that web developers use to increase interactivity and boost the dynamic experience for the users. According to Statista, nearly 65% of software developers worldwide use JavaScript for webpage development.
JavaScript is an indispensable part of the modern internet. Web3Techs reports that 95% of websites use JavaScript and is one of the most popular programming languages in the world. In addition, it is versatile, beginner-friendly, compact, and highly flexible, making it easier for web developers to write a variety of tools on top of the JavaScript language.
What Is JavaScript?
JavaScript is a client-side script or programming language that allows you to implement complex features on web pages, such as:
- Image scrolling
- Web page loading without refreshing the page
- Dropdown menus
- Animated 2D/3D images
- Scrolling videos
- Interactive maps
- Playing audio and videos
- Autocomplete
One of JavaScript’s most powerful functions is asynchronous interaction with a remote server. The programming language communicates with the server in the background without interrupting the user interaction. So, for example, when a user types in “buy shoes,” a list of possible suggestions like “buy shoes online,” “buy shoes USA,” “buy shoes near me” comes up.
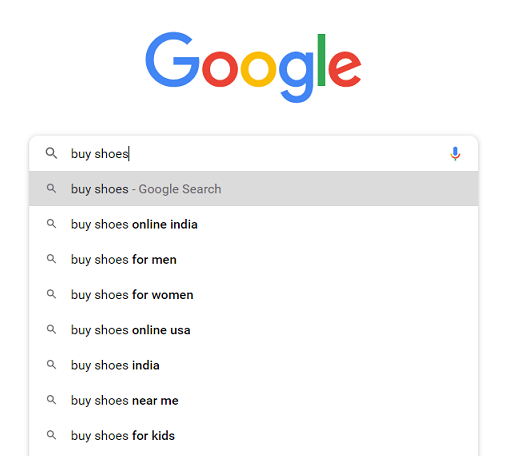
This is because JavaScript reads the letter than the user types and sends it to a remote server that sends the autocomplete suggestion back. The programming language’s function on the user’s machine is as simple as possible not to slow down the user’s experience.
Before JavaScript, web pages provided users with little to no interaction options, restricting clients’ actions to only loading pages and clicking links. But with the development of JavaScript in the late 1990s, web pages could include animations and other forms of interactive content.
How Does JavaScript Function?
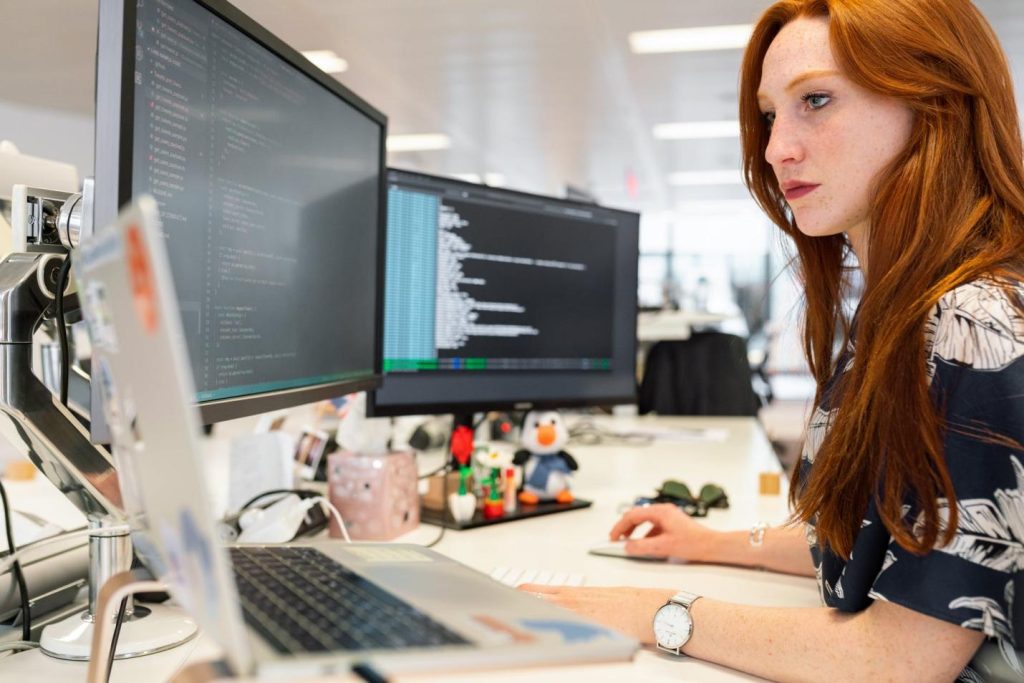
When you use any web application, there is an interaction between the user’s device and the remote server. So, for example, when you search for anything on the search engine, the remote server software sends the required information to the client’s software which reads the data and loads the necessary web page on the user’s screen.
However, the programming language JavaScript performs all functions on the client’s device and doesn’t have to interact with the remote server. So, for example, when you have a web page loaded on your device, and the internet goes down all of a sudden, you would still be able to view the web page as long as you don’t refresh it.
JavaScript works with HTML, CSS and is compatible with modern web browsers like Google Chrome, Internet Explorer, Firefox, Opera, Microsoft Edge, etc. When a web browser loads a page, it parses the HTML and creates the Document Object Model (DOM), which presents a live view of the web page to the JavaScript code.
The browser stores everything related to the HTML, such as images and CSS files. Then, the DOM combines the HTML and CSS together to create the web page. Once the JavaScript’s engine loads the JavaScript files and inline codes, it does not run it immediately but waits for the HTML and CSS to finish loading.
Once complete, the software executes the JavaScript program in the order of the written code. This helps the code update the DOM and enables the browser to render it.
7 JavaScript Concepts That All Web Developers Need To Know
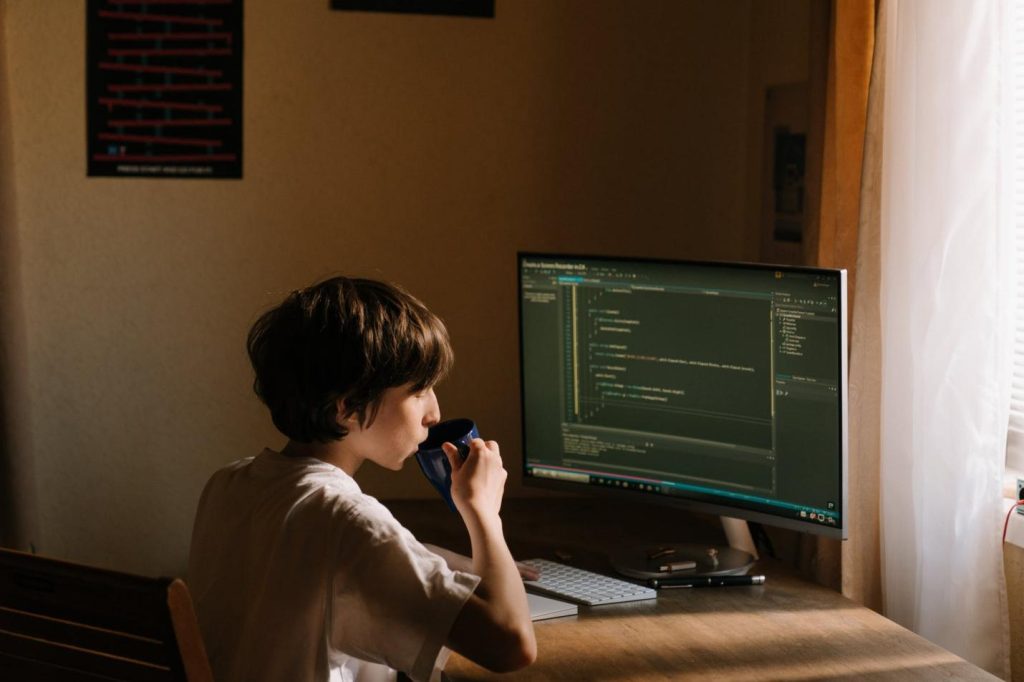
With the world of web development moving rapidly, it is vital to ensure that learning JavaScript can benefit you in the long run. With the 2021 StackOverflow developer survey crowning JavaScript as the most commonly used programming language for the ninth time in a row, you needn’t fear the relevancy of mastering this script.
Currently, more than 90% of websites worldwide use JavaScript in one form or another to improve the user experience and increase interactivity. Moreover, proficiency in this language enables you to build websites from scratch – a skill with fantastic job opportunities in the current market.
So, let’s dive into the 7 essential JavaScript concepts that you should know if you wish to master the script:
1. JavaScript Scope
In JavaScript, Scope is the current code context that determines the accessibility of the variables and other resources to JavaScript. There are two types of scope that you will come across:
- Global variables that are declared outside the block
- Local variables that are declared inside the block
Now, let’s assume you want to create a function and access a variable defined in the global scope. So, let’s create a global variable:
// Initialize a global variable
var creature = “wolf”;
Now, using a local scope, you can create new variables with the same name as that in the outer scope without changing or reassigning the original value.
2. JavaScript Closures
In JavaScript, a closure combines the function and the lexical environment in which the user developer declares the function. It is an inner function that can access the outer enclosing function’s variables. You can use closure to extend a behaviour, such as passing variables from an outer function to an inner function. Additionally, it is helpful whenever you have to work with events as you can access the context defined in the outer function from the inner function.
The closure has three scope chains:
- It can access its own scope, that is, the variables defined in the curly brackets
- It can access the outer function’s variables
- It can access the global variables
Consider the following code for example:
function makeFunc() {
var name = 'Mozilla';
function displayName() {
alert(name);
}
return displayName;
}
var myFunc = makeFunc();
myFunc();
When you run this code, the outer function outer function makeFunc() returns an inner function as displayName() before it is executed.
3. Hoisting
Hoisting in JavaScript is the process where you can move variables and function declarations to the top of their scope before the code execution. It ensures that no matter where you declare the functions and variables in the code, they will automatically move on top of the scope regardless of whether it is global or local. Therefore, you can use a function or variable before the declaration.
For example,
// using test before declaring
console.log(test); // undefined
var test;
The output of this program is undefined as it behaves as:
// using test before declaring
var test;
console.log(test); // undefin
Since the variable test is declared yet has no value, the value undefined is attached to it.
During hosting, you should keep in mind that even though the declaration moves up in the program, the function and variable declarations get added to the compile phase memory.
4. Memoization
Memoization is the optimisation process where the program increases the function’s performance by caching the previous computed results. JavaScript’s ability to behave as associative arrays makes it the perfect candidate to act as caches. The necessity for speed when carrying out heavy computations makes memoization a vital function. An example of this is:
// a simple function to add something
const add = (n) => (n + 10);
add(9);
// a simple memoized function to add something
const memoizedAdd = () => {
let cache = {};
return (n) => {
if (n in cache) {
console.log('Fetching from cache');
return cache[n];
}
else {
console.log('Calculating result');
let result = n + 10;
cache[n] = result;
return result;
}
}
}
// returned function from memoizedAdd
const newAdd = memoizedAdd();
console.log(newAdd(9)); // calculated
console.log(newAdd(9)); // cached
5. The Module Pattern
A module is a small unit of independent, reusable code that is the foundation of various JavaScript design patterns. It is also vital when you need to build a non-trivial JavaScript-based application.
Additionally, you can use a module pattern to wrap up a set of variables and functions together in a single scope. The benefits of this module pattern are:
- Maintainability – Module pattern ensures better maintainability because every code is encapsulated in a single logical block. This makes it easier to update the independent blocks.
- Reusability – Module pattern also allows you to reuse a single unit of code across the entire platform. In addition, since you can reuse the functionality enclosed in the module multiple times, you won’t have to define the same functions repeatedly.
6. IIFE
IIFE, also known as the Immediately Invoked Function Expression, is a popular design pattern in JavaScript. The programming script allows you to define variables and functions inside a function that you cannot access outside of it. However, sometimes, a problem arises when you might accidentally pollute the global functions or variables by using the same name.
However, IIFE resolves this issue by having its own scope, restricting functions and variables to become global. In such cases, the functions and variables you declare inside the IIFE will be unable to pollute the global scope despite having the same global functions and variables.
7. Polymorphism
Polymorphism is a tenet of Object-Oriented Programming (OOP) that allows you to perform a single action in different forms. It also permits you to call the same method on other JavaScript objects, making it possible to design objects so that they can override any behaviour with the specified provided objects.
For example:
<script>
class A {
display()
{
document.writeln("A is invoked");
}
}
class B extends A
{
}
var b=new B();
b.display();
</script>
Here, the output is:
A is invoked
Thus, you can see how the child class object invokes the parent class method.
Summing it up,
As JavaScript is one of the most popular programming languages globally, there’s no doubt that it is a highly sought-after skill in the web development industry. Unfortunately, the demand outweighs the supply of adept people in this programming language. If you’re planning to explore JavaScript, mastering the seven concepts in this blog can help you immensely. As it is a beginner-friendly language that requires minimal coding, you can expect to see results soon.
Author bio:
Diana Rosell is a professional academic expert at MyAssignmenthelp.com, providing IT assistance regarding thesis statements to students in the UK, US and Canada. After completing his Masters from a reputed university in the UK, she plans to continue researching the latest IT trends worldwide. In addition, Rosell loves to spend her weekends with his family going camping.
SIEM at a glance (Security information and event management)
You have a contingency plan like most other intelligent businesses, but what if it fails? And,…
0 Comments11 Minutes
Breaking the Mold: Unconventional Methods for Business Growth with WordPress
If you have implemented WordPress for your website, or are considering migrating to it, chances…
0 Comments13 Minutes
Web and Mobile App Development Trends to Consider in 2024
As technology continues to evolve at a rapid pace, staying ahead is essential for developers and…
0 Comments8 Minutes
What Are the Top 10 Web Development Tools for Beginners?
Web development is the art of building and updating web pages that Internet users Interact with.…
0 Comments13 Minutes
Does Your Branding Need A New Look?
From time to time, it’s important and necessary to check over your branding, to ensure that it is…
0 Comments4 Minutes
Essential Skills for AI-Driven Digital Marketing
Digital marketing is changing a lot. Artificial intelligence (AI) is becoming really powerful and…
0 Comments10 Minutes
A Handbook for Crafting Outstanding Email Automation Plans
Email marketing is an essential tool for communication in digital marketing platforms. Managing…
0 Comments8 Minutes
Branding – What It Means for Your Business
Every single one of us has our own personal brand. Unique to each of us, this lies in who we are…
0 Comments5 Minutes
13 Comments
Comments are closed.
Really very happy to say, your post is very interesting to read.
Your Post is very useful, I am truly happy to post my note on this blog. It helped me a lot..worked
Thanks for sharing your opinion hope you will share more post.
Thanks! I am glad that it helped.
Thanks for sharing your opinion hope you will share more post.
Essentially Fantastic, Thank you for this valuable information. I appreciate this blog.
Thank you to provide us this useful information.
Your all blog is amazing because i am regular reader of you blogs
Thanks for sharing
Thank you to provide us this useful information.
Your all blog is amazing Thanks for sharing.
Very Interesting Post, thanks for sharing.
I am thankful for the article post.Looking forward to visit more.
Thanks for sharing this information.The survey